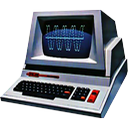
index.include.php
<?php
// this file goes in each page's directory and includes the global
// index rendering script
// user's root webserver directory
$userRootDir = 'Sites';
// check if site is in server root or user directory
$uri = $_SERVER[ 'REQUEST_URI' ];
$uid = posix_getpwuid( fileowner( __FILE__ ) );
$userDir = '~'.$uid[ 'name'];
// make include filepath based on site location
if( stristr( $uri, $userDir ) )
{
$index = $uid[ 'dir' ].'/'.$userRootDir.'/lib/index.render.php';
}
else
{
$index = $_SERVER[ 'DOCUMENT_ROOT' ].'/lib/index.render.php';
}
// include index for this directory
require( $index );
?>
// this file goes in each page's directory and includes the global
// index rendering script
// user's root webserver directory
$userRootDir = 'Sites';
// check if site is in server root or user directory
$uri = $_SERVER[ 'REQUEST_URI' ];
$uid = posix_getpwuid( fileowner( __FILE__ ) );
$userDir = '~'.$uid[ 'name'];
// make include filepath based on site location
if( stristr( $uri, $userDir ) )
{
$index = $uid[ 'dir' ].'/'.$userRootDir.'/lib/index.render.php';
}
else
{
$index = $_SERVER[ 'DOCUMENT_ROOT' ].'/lib/index.render.php';
}
// include index for this directory
require( $index );
?>
index.render.php
<?php
require( 'main.php' );
global $docRoot;
global $userRoot;
$description = file_get_contents( 'files/metadata/description.txt' );
$keywords = file_get_contents( 'files/metadata/keywords.txt' );
$title = file_get_contents( 'files/metadata/title.txt' );
$copyright = '(c)(p) '.date( 'Y' ).' Cooper Baker - All Rights Reserved';
$timestamp = '<!-- This page was automatically generated on '.date( 'l F jS Y' ).' at '.date( 'g:i:sa' )." -->\n";
$stylesheet = $userRoot.'/lib/style.php';
$favicon = $userRoot.'/lib/images/favicon.png';
?>
<!DOCTYPE html>
<html>
<head>
<?php echo $timestamp; ?>
<meta charset="UTF-8">
<meta name="author" content="Cooper Baker">
<meta name="copyright" content="<?php echo $copyright; ?>">
<meta name="description" content="<?php echo $description; ?>">
<meta name="keywords" content="<?php echo $keywords; ?>">
<link rel="stylesheet" href="<?php echo $stylesheet; ?>" type="text/css">
<link rel="icon" href="<?php echo $favicon; ?>" type="image/x-icon" type="text/css">
<title><?php echo $title; ?></title>
<meta name="robots" content="index, follow">
<meta name="googlebot" content="index, follow">
<?php require( $docRoot.'/lib/google.verification.php' ); ?>
</head>
<body>
<div id="menu">
<div id="menuleft">
<?php echo $title; ?>
</div>
<div id="menuright">
<?php breadcrumbs(); ?>
</div>
</div>
<br>
<div id="content">
<?php require( 'files/content.php' ); ?>
<br>
<br>
</div>
<div id="copyright">
(c)(p)<?php echo date( 'Y' ); ?> Cooper Baker
·
All Rights Reserved
·
<?php echo hitCount( 'files/metadata/hits.txt' ); ?>
·
<?php echo hitCount( $docRoot.'/lib/hits.txt' ); ?>
</div>
<?php require( $docRoot.'/lib/google.analytics.php' ); ?>
</body>
</html>
<?php visitorLog() ?>
require( 'main.php' );
global $docRoot;
global $userRoot;
$description = file_get_contents( 'files/metadata/description.txt' );
$keywords = file_get_contents( 'files/metadata/keywords.txt' );
$title = file_get_contents( 'files/metadata/title.txt' );
$copyright = '(c)(p) '.date( 'Y' ).' Cooper Baker - All Rights Reserved';
$timestamp = '<!-- This page was automatically generated on '.date( 'l F jS Y' ).' at '.date( 'g:i:sa' )." -->\n";
$stylesheet = $userRoot.'/lib/style.php';
$favicon = $userRoot.'/lib/images/favicon.png';
?>
<!DOCTYPE html>
<html>
<head>
<?php echo $timestamp; ?>
<meta charset="UTF-8">
<meta name="author" content="Cooper Baker">
<meta name="copyright" content="<?php echo $copyright; ?>">
<meta name="description" content="<?php echo $description; ?>">
<meta name="keywords" content="<?php echo $keywords; ?>">
<link rel="stylesheet" href="<?php echo $stylesheet; ?>" type="text/css">
<link rel="icon" href="<?php echo $favicon; ?>" type="image/x-icon" type="text/css">
<title><?php echo $title; ?></title>
<meta name="robots" content="index, follow">
<meta name="googlebot" content="index, follow">
<?php require( $docRoot.'/lib/google.verification.php' ); ?>
</head>
<body>
<div id="menu">
<div id="menuleft">
<?php echo $title; ?>
</div>
<div id="menuright">
<?php breadcrumbs(); ?>
</div>
</div>
<br>
<div id="content">
<?php require( 'files/content.php' ); ?>
<br>
<br>
</div>
<div id="copyright">
(c)(p)<?php echo date( 'Y' ); ?> Cooper Baker
·
All Rights Reserved
·
<?php echo hitCount( 'files/metadata/hits.txt' ); ?>
·
<?php echo hitCount( $docRoot.'/lib/hits.txt' ); ?>
</div>
<?php require( $docRoot.'/lib/google.analytics.php' ); ?>
</body>
</html>
<?php visitorLog() ?>
main.php
<?php
// turn on error reporting
error_reporting( E_ALL ^ E_NOTICE );
ini_set( 'display_errors', 1 );
// set timezone
date_default_timezone_set( 'America/Los_Angeles' );
// includes
require( 'render.php' );
require( 'utility.php' );
// get root directory for files
$docRoot = realpath( __DIR__.'/../' );
// get URI root directory of user
$userRoot = userRootDir();
?>
// turn on error reporting
error_reporting( E_ALL ^ E_NOTICE );
ini_set( 'display_errors', 1 );
// set timezone
date_default_timezone_set( 'America/Los_Angeles' );
// includes
require( 'render.php' );
require( 'utility.php' );
// get root directory for files
$docRoot = realpath( __DIR__.'/../' );
// get URI root directory of user
$userRoot = userRootDir();
?>
render.php
<?php
//-----------------------------------------------------------------------------
// gallery -
// draws a grid of clickable thumbnails from a directory containing
// a 'thumbs' and 'images' subdirectory with matching file names in each
// with a thin border by default
//-----------------------------------------------------------------------------
function gallery( $dir = 'gallery', $border = 1 )
{
global $docRoot;
global $userRoot;
$dir = 'files/'.$dir;
$ignore = array( '.', '..', '.DS_Store' );
$dh = opendir( $dir.'/thumbs' );
$column = 0;
$path = '';
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
if( !in_array( $file, $ignore ) )
{
if( $column == 0 )
{
echo '<div class="gallery">';
}
$path = realpath( '.' );
$path = $path.'/'.$dir.'/images/'.$file;
$path = str_replace( $docRoot, $userRoot, $path );
echo '<div class="thumbBox">';
echo '<a href="'.$userRoot.'/lib/view.php?image='.$path.'" target="_blank">';
if( $border )
{
echo '<img src="'.$dir.'/thumbs/'.$file.'" class="thumb">';
}
else
{
echo '<img src="'.$dir.'/thumbs/'.$file.'">';
}
echo '<br>';
echo substr( $file, 0, -4 );
echo '</a>';
echo '</div>';
$column++;
if( $column > 3 )
{
echo '</div>';
$column = 0;
}
}
}
if( ( $column > 0 ) && ( $column <= 3 ) )
{
echo '</div>';
}
}
//-----------------------------------------------------------------------------
// code - draws colorized source code from $file using geshi
//-----------------------------------------------------------------------------
function code( $file, $type = '' )
{
global $docRoot;
include_once( 'geshi/geshi.php' );
$file = 'files/'.$file;
$source = file_get_contents( $file );
$ext = pathinfo( $file, PATHINFO_EXTENSION );
$path = $docRoot.'/lib/geshi/';
if( $type )
{
$geshi = new GeSHi( $source, $type );
}
else
{
$geshi = new GeSHi( $source, $ext );
}
$geshi->enable_classes();
$geshi->enable_keyword_links( false );
$geshi->set_header_type( GESHI_HEADER_DIV );
echo "<style>\n";
echo $geshi->get_stylesheet();
echo "</style>\n";
echo '<div class="code">';
echo $geshi->parse_code();
echo '</div>';
unset( $geshi );
}
//-----------------------------------------------------------------------------
// github - draws colorized source code from gihub raw $url using geshi
//-----------------------------------------------------------------------------
function github( $url, $type = '' )
{
global $docRoot;
include_once( 'geshi/geshi.php' );
$source = file_get_contents( $url );
$ext = pathinfo( $url, PATHINFO_EXTENSION );
$path = $docRoot.'/lib/geshi/';
if( $type )
{
$geshi = new GeSHi( $source, $type );
}
else
{
$geshi = new GeSHi( $source, $ext );
}
$geshi->enable_classes();
$geshi->enable_keyword_links( false );
$geshi->set_header_type( GESHI_HEADER_DIV );
error_reporting( E_ALL ^ E_DEPRECATED );
echo "<style>\n";
echo $geshi->get_stylesheet();
echo "</style>\n";
echo '<div class="code">';
echo $geshi->parse_code();
echo '</div>';
unset( $geshi );
}
//-----------------------------------------------------------------------------
// ruler - draws a ruler with $title
//-----------------------------------------------------------------------------
function ruler( $title = '' )
{
echo '<div class="ruler">';
echo $title;
echo '</div>';
}
//-----------------------------------------------------------------------------
// bigText - draws big $text
//-----------------------------------------------------------------------------
function bigText( $text )
{
echo '<span class="bigText">'.$text.'</span>';
}
//-----------------------------------------------------------------------------
// text - draws $text
//-----------------------------------------------------------------------------
function text( $text )
{
echo '<div class="text">';
echo $text;
echo '</div>';
}
//-----------------------------------------------------------------------------
// image - draws $file
//-----------------------------------------------------------------------------
function image( $file, $width = '' )
{
$file = 'files/'.$file;
echo '<div class="image">';
if( $width )
{
echo '<img src="'.$file.'" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'">';
}
echo '</div>';
}
//-----------------------------------------------------------------------------
// photo - draws $file
//-----------------------------------------------------------------------------
function photo( $file, $width = '' )
{
$file = 'files/'.$file;
echo '<div class="photoBox">';
if( $width )
{
echo '<img src="'.$file.'" class="photo" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="photo">';
}
echo '</div>';
}
//-----------------------------------------------------------------------------
// audio - draws an audio player for $file of type .mp3 or .wav
//-----------------------------------------------------------------------------
function audio( $file, $preload = 1 )
{
$temp = explode( "/", $file );
$temp = $temp[ count( $temp ) - 1 ];
$temp = explode( ".", $temp );
$name = $temp[ 0 ];
$ext = pathinfo( $file, PATHINFO_EXTENSION );
$file = 'files/'.$file;
echo '<div class="audio">';
if( $preload )
{
echo '<audio controls class="audioPlayer">';
}
else
{
echo '<audio controls class="audioPlayer" preload="none">';
}
switch( $ext )
{
case "mp3" : echo '<source src="'.$file.'" type="audio/mpeg">';
break;
case "wav" : echo '<source src="'.$file.'" type="audio/wav">';
break;
}
echo '<a href="'.$file.'">'.$name.'.'.$ext.'</a>';
echo '</audio>';
echo '</div>';
}
//-----------------------------------------------------------------------------
// children - draws links and descriptions to child directories
//-----------------------------------------------------------------------------
function children()
{
$ignore = array( '.', '..', '.DS_Store', 'files', 'geshi', 'temp' );
$dh = @opendir( '.' );
$descrip = '';
echo '<nav>';
echo '<div class="children">';
echo '<table class="childTable">';
while( $dir = readdir( $dh ) )
{
$dirs[] = $dir;
}
closedir( $dh );
natsort( $dirs );
foreach( $dirs as $dir )
{
if( !in_array( $dir, $ignore ) )
{
if( is_dir( $dir ) )
{
$title = file_get_contents( $dir.'/files/metadata/title.txt' );
$descrip = file_get_contents( $dir.'/files/metadata/description.txt' );
echo '<tr>';
echo '<td class="childLink">';
echo '<a href="'.$dir.'" class="child">'.$title.'</a>';
echo '</td>';
echo '<td class="childDescription">';
// echo ' '.$descrip;
echo $descrip;
echo '</td>';
echo '</tr>';
}
}
}
echo '</table>';
echo '</div>';
echo '</nav>';
}
//-----------------------------------------------------------------------------
// childrenIcons - draws icons, links, and descriptions to child directories
//-----------------------------------------------------------------------------
function childrenIcons()
{
$ignore = array( '.', '..', '.DS_Store', 'files', 'geshi', 'temp' );
$dh = @opendir( '.' );
$descrip = '';
$icon = '';
echo '<nav>';
echo '<div class="children">';
echo '<table class="childTable">';
while( $dir = readdir( $dh ) )
{
$dirs[] = $dir;
}
closedir( $dh );
natsort( $dirs );
foreach( $dirs as $dir )
{
if( !in_array( $dir, $ignore ) )
{
if( is_dir( $dir ) )
{
$title = file_get_contents( $dir.'/files/metadata/title.txt' );
$descrip = file_get_contents( $dir.'/files/metadata/description.txt' );
$icon = $dir.'/files/metadata/icon.png';
echo '<tr>';
echo '<td class="childIcon" rowspan="2">';
echo '<a href="'.$dir.'" class="child">';
echo '<img src="'.$icon.'" class="icon">';
echo '</a>';
echo '</td>';
echo '<td class="childIconLink">';
echo '<a href="'.$dir.'" class="child">'.$title.'</a>';
echo '</td>';
echo '</tr>';
echo '<tr>';
echo '<td class="childIconDescription">';
echo $descrip;
echo '</td>';
echo '</tr>';
}
}
}
echo '</table>';
echo '</div>';
echo '</nav>';
}
//-----------------------------------------------------------------------------
// video - draws a video player for $file of type mp4/aac (.m4v)
//-----------------------------------------------------------------------------
function video( $file )
{
$temp = explode( "/", $file );
$name = $temp[ count( $temp ) - 1 ];
$file = 'files/'.$file;
echo '<div class="video">';
echo '<video width="640" height="480" controls class="videoPlayer"';
echo '<source src="'.$file.'" type="video/mp4">';
echo '<a href="'.$file.'">'.$name.'</a>';
echo '</video>';
echo '</div>';
}
//-----------------------------------------------------------------------------
// href - draws a link pointing to $url with the name $title
//-----------------------------------------------------------------------------
function href( $url, $title )
{
echo '<a href="'.$url.'" target="_blank">'.$title.'</a>';
}
//-----------------------------------------------------------------------------
// download - draws a link for download pointing to $file with the name $title
//-----------------------------------------------------------------------------
function download( $file, $title )
{
$file = 'files/'.$file;
echo '<a href="'.$file.'" target="_blank">'.$title.'</a>';
}
//-----------------------------------------------------------------------------
// includeFile - includes $file onto the current page
//-----------------------------------------------------------------------------
function includeFile( $file )
{
$file = 'files/'.$file;
require( $file );
}
//-----------------------------------------------------------------------------
// embed - loads $url into a string then draws it onto the current page
//-----------------------------------------------------------------------------
function embed( $url )
{
$string = file_get_contents( $url );
echo $string;
}
//-----------------------------------------------------------------------------
// scrapeBody - scrapes the body out of an html document and echoes it locally
//-----------------------------------------------------------------------------
function scrapeBody( $url )
{
$pagestring = file_get_contents( $url );
$pagelength = strlen( $pagestring );
$bodyopen = strpos( $pagestring, '<body' );
$bodyclose = strpos( $pagestring, '</body>' );
$bodystring = substr( $pagestring, $bodyopen, ( $bodyclose - $pagelength ) );
$bodystring = substr( $bodystring, strpos( $bodystring, '>' ) + 1 );
echo $bodystring;
}
//-----------------------------------------------------------------------------
// breadcrumbs - draws links that lead back to the home page
//-----------------------------------------------------------------------------
function breadcrumbs()
{
global $userRoot;
$file = $_SERVER[ 'PHP_SELF' ];
$dir = pathinfo( $file, PATHINFO_DIRNAME );
$path = str_replace( $userRoot, '', $dir );
$dirs = explode( '/', $path );
$size = count( $dirs ) - 2;
$url = '';
echo '<div class="breadcrumbs">';
echo ' ';
echo '← ';
for( $i = $size; $i > 0 ; $i-- )
{
$url .= '../';
echo '<a href="'.$url.'">'.$dirs[ $i ].'</a> · ';
}
echo '<a href="'.$userRoot.'/home/search">search</a>';
echo '</div>';
}
//-----------------------------------------------------------------------------
// sitemap - draws a list of links to each page
//-----------------------------------------------------------------------------
function sitemap( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$ignore = array( '.', '..', '.DS_Store', 'files', 'Temp' );
if( $depth == 0 )
{
$dir = $docRoot.'/home';
echo '<a href="'.$userRoot.'/home">home</a><br>';
$depth = 1;
}
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
if( is_dir( $dir.'/'.$file ) && !in_array( $file, $ignore ) )
{
$url = $dir.'/'.$file;
$url = str_replace( $docRoot, $userRoot, $url );
echo str_repeat( ' ', ( $depth * 4 ) );
echo '<a href="'.$url.'">'.$file.'</a><br>';
sitemap( $dir.'/'.$file, ( $depth + 1 ) );
}
}
}
//-----------------------------------------------------------------------------
// sitemapEdit - draws a list of links to each page with query strings for edit
//-----------------------------------------------------------------------------
function sitemapEdit( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$ignore = array( '.', '..', '.DS_Store', 'files', 'Under Construction' );
if( $depth == 0 )
{
$dir = $docRoot.'/home';
echo '<a href="index.php?path='.$dir.'">home</a><br>';
$depth = 1;
}
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
$path = $dir.'/'.$file;
if( is_dir( $path ) && !in_array( $file, $ignore ) )
{
echo str_repeat( ' ', ( $depth * 4 ) );
echo '<a href="index.php?path='.$path.'">'.$file.'</a><br>';
sitemapEdit( $path, ( $depth + 1 ) );
}
}
}
//-----------------------------------------------------------------------------
// listFilesEdit - draws a list of files in each page's files directory
//-----------------------------------------------------------------------------
function listFilesEdit( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$ignore = array( '.', '..', '.DS_Store', 'metadata', 'content.php' );
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
$path = $dir.'/'.$file;
if( !in_array( $file, $ignore ) )
{
echo str_repeat( ' ', ( $depth * 4 ) );
if( is_dir( $path ) )
{
echo $file.'<br>';
listFilesEdit( $path, ( $depth + 1 ) );
}
else
{
$url = str_replace( $docRoot, $userRoot, $path );
echo '<a href="'.$url.'" target="_blank">'.$file.'</a><br>';
}
}
}
}
//-----------------------------------------------------------------------------
// googleMap - uses google maps api to draw a map centered at $lat, $lon
//-----------------------------------------------------------------------------
function googleMap( $lat, $lon )
{
echo '<div id="googleMapBox">';
echo ' <div id="googleMap">';
echo ' </div>';
echo '</div>';
echo '<script src="https://maps.googleapis.com/maps/api/js?v=3.exp&sensor=false"></script>';
echo '<script>';
echo ' function initialize()';
echo ' {';
echo ' var latLon = new google.maps.LatLng( '.$lat.', '.$lon.' );';
echo ' var mapOptions =';
echo ' {';
echo ' zoom: 10,';
echo ' center: latLon,';
echo ' disableDefaultUI: true,';
echo ' draggable: false,';
echo ' scrollwheel: false';
echo ' };';
echo ' var map = new google.maps.Map(document.getElementById( "googleMap" ), mapOptions );';
echo ' var marker = new google.maps.Marker( { position: latLon, map: map } );';
echo ' infowindow.open( map, marker );';
echo ' }';
echo ' initialize();';
echo '</script>';
}
//-----------------------------------------------------------------------------
// dropImage - drops a left floating image for text to flow around
//-----------------------------------------------------------------------------
function dropImage( $file, $width = '' )
{
$file = 'files/'.$file;
if( $width )
{
echo '<img src="'.$file.'" class="dropImage" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="dropImage">';
}
}
//-----------------------------------------------------------------------------
// dropPhoto - drops a left floating photo for text to flow around
//-----------------------------------------------------------------------------
function dropPhoto( $file, $width = '' )
{
$file = 'files/'.$file;
if( $width )
{
echo '<img src="'.$file.'" class="dropPhoto" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="dropPhoto">';
}
}
//-----------------------------------------------------------------------------
// dropIcon - drops a left floating icon for text to flow around
// circular icons should have a 2px black border
//-----------------------------------------------------------------------------
function dropIcon( $file = 'icon.png', $width = '' )
{
$file = 'files/metadata/'.$file;
if( $width )
{
echo '<img src="'.$file.'" class="dropPhoto" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="dropIcon">';
}
}
//-----------------------------------------------------------------------------
// ls - draws a list of files
//-----------------------------------------------------------------------------
function ls( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$dir = 'files/'.$dir;
$ignore = array( '.', '..', '.DS_Store', 'metadata', 'content.php' );
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
$path = $dir.'/'.$file;
if( !in_array( $file, $ignore ) )
{
echo str_repeat( ' ', ( $depth * 4 ) );
if( is_dir( $path ) )
{
echo $file.'<br>';
listFilesEdit( $path, ( $depth + 1 ) );
}
else
{
$url = str_replace( $docRoot, $userRoot, $path );
echo '<a href="'.$url.'" target="_blank">'.$file.'</a><br>';
}
}
}
}
?>
//-----------------------------------------------------------------------------
// gallery -
// draws a grid of clickable thumbnails from a directory containing
// a 'thumbs' and 'images' subdirectory with matching file names in each
// with a thin border by default
//-----------------------------------------------------------------------------
function gallery( $dir = 'gallery', $border = 1 )
{
global $docRoot;
global $userRoot;
$dir = 'files/'.$dir;
$ignore = array( '.', '..', '.DS_Store' );
$dh = opendir( $dir.'/thumbs' );
$column = 0;
$path = '';
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
if( !in_array( $file, $ignore ) )
{
if( $column == 0 )
{
echo '<div class="gallery">';
}
$path = realpath( '.' );
$path = $path.'/'.$dir.'/images/'.$file;
$path = str_replace( $docRoot, $userRoot, $path );
echo '<div class="thumbBox">';
echo '<a href="'.$userRoot.'/lib/view.php?image='.$path.'" target="_blank">';
if( $border )
{
echo '<img src="'.$dir.'/thumbs/'.$file.'" class="thumb">';
}
else
{
echo '<img src="'.$dir.'/thumbs/'.$file.'">';
}
echo '<br>';
echo substr( $file, 0, -4 );
echo '</a>';
echo '</div>';
$column++;
if( $column > 3 )
{
echo '</div>';
$column = 0;
}
}
}
if( ( $column > 0 ) && ( $column <= 3 ) )
{
echo '</div>';
}
}
//-----------------------------------------------------------------------------
// code - draws colorized source code from $file using geshi
//-----------------------------------------------------------------------------
function code( $file, $type = '' )
{
global $docRoot;
include_once( 'geshi/geshi.php' );
$file = 'files/'.$file;
$source = file_get_contents( $file );
$ext = pathinfo( $file, PATHINFO_EXTENSION );
$path = $docRoot.'/lib/geshi/';
if( $type )
{
$geshi = new GeSHi( $source, $type );
}
else
{
$geshi = new GeSHi( $source, $ext );
}
$geshi->enable_classes();
$geshi->enable_keyword_links( false );
$geshi->set_header_type( GESHI_HEADER_DIV );
echo "<style>\n";
echo $geshi->get_stylesheet();
echo "</style>\n";
echo '<div class="code">';
echo $geshi->parse_code();
echo '</div>';
unset( $geshi );
}
//-----------------------------------------------------------------------------
// github - draws colorized source code from gihub raw $url using geshi
//-----------------------------------------------------------------------------
function github( $url, $type = '' )
{
global $docRoot;
include_once( 'geshi/geshi.php' );
$source = file_get_contents( $url );
$ext = pathinfo( $url, PATHINFO_EXTENSION );
$path = $docRoot.'/lib/geshi/';
if( $type )
{
$geshi = new GeSHi( $source, $type );
}
else
{
$geshi = new GeSHi( $source, $ext );
}
$geshi->enable_classes();
$geshi->enable_keyword_links( false );
$geshi->set_header_type( GESHI_HEADER_DIV );
error_reporting( E_ALL ^ E_DEPRECATED );
echo "<style>\n";
echo $geshi->get_stylesheet();
echo "</style>\n";
echo '<div class="code">';
echo $geshi->parse_code();
echo '</div>';
unset( $geshi );
}
//-----------------------------------------------------------------------------
// ruler - draws a ruler with $title
//-----------------------------------------------------------------------------
function ruler( $title = '' )
{
echo '<div class="ruler">';
echo $title;
echo '</div>';
}
//-----------------------------------------------------------------------------
// bigText - draws big $text
//-----------------------------------------------------------------------------
function bigText( $text )
{
echo '<span class="bigText">'.$text.'</span>';
}
//-----------------------------------------------------------------------------
// text - draws $text
//-----------------------------------------------------------------------------
function text( $text )
{
echo '<div class="text">';
echo $text;
echo '</div>';
}
//-----------------------------------------------------------------------------
// image - draws $file
//-----------------------------------------------------------------------------
function image( $file, $width = '' )
{
$file = 'files/'.$file;
echo '<div class="image">';
if( $width )
{
echo '<img src="'.$file.'" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'">';
}
echo '</div>';
}
//-----------------------------------------------------------------------------
// photo - draws $file
//-----------------------------------------------------------------------------
function photo( $file, $width = '' )
{
$file = 'files/'.$file;
echo '<div class="photoBox">';
if( $width )
{
echo '<img src="'.$file.'" class="photo" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="photo">';
}
echo '</div>';
}
//-----------------------------------------------------------------------------
// audio - draws an audio player for $file of type .mp3 or .wav
//-----------------------------------------------------------------------------
function audio( $file, $preload = 1 )
{
$temp = explode( "/", $file );
$temp = $temp[ count( $temp ) - 1 ];
$temp = explode( ".", $temp );
$name = $temp[ 0 ];
$ext = pathinfo( $file, PATHINFO_EXTENSION );
$file = 'files/'.$file;
echo '<div class="audio">';
if( $preload )
{
echo '<audio controls class="audioPlayer">';
}
else
{
echo '<audio controls class="audioPlayer" preload="none">';
}
switch( $ext )
{
case "mp3" : echo '<source src="'.$file.'" type="audio/mpeg">';
break;
case "wav" : echo '<source src="'.$file.'" type="audio/wav">';
break;
}
echo '<a href="'.$file.'">'.$name.'.'.$ext.'</a>';
echo '</audio>';
echo '</div>';
}
//-----------------------------------------------------------------------------
// children - draws links and descriptions to child directories
//-----------------------------------------------------------------------------
function children()
{
$ignore = array( '.', '..', '.DS_Store', 'files', 'geshi', 'temp' );
$dh = @opendir( '.' );
$descrip = '';
echo '<nav>';
echo '<div class="children">';
echo '<table class="childTable">';
while( $dir = readdir( $dh ) )
{
$dirs[] = $dir;
}
closedir( $dh );
natsort( $dirs );
foreach( $dirs as $dir )
{
if( !in_array( $dir, $ignore ) )
{
if( is_dir( $dir ) )
{
$title = file_get_contents( $dir.'/files/metadata/title.txt' );
$descrip = file_get_contents( $dir.'/files/metadata/description.txt' );
echo '<tr>';
echo '<td class="childLink">';
echo '<a href="'.$dir.'" class="child">'.$title.'</a>';
echo '</td>';
echo '<td class="childDescription">';
// echo ' '.$descrip;
echo $descrip;
echo '</td>';
echo '</tr>';
}
}
}
echo '</table>';
echo '</div>';
echo '</nav>';
}
//-----------------------------------------------------------------------------
// childrenIcons - draws icons, links, and descriptions to child directories
//-----------------------------------------------------------------------------
function childrenIcons()
{
$ignore = array( '.', '..', '.DS_Store', 'files', 'geshi', 'temp' );
$dh = @opendir( '.' );
$descrip = '';
$icon = '';
echo '<nav>';
echo '<div class="children">';
echo '<table class="childTable">';
while( $dir = readdir( $dh ) )
{
$dirs[] = $dir;
}
closedir( $dh );
natsort( $dirs );
foreach( $dirs as $dir )
{
if( !in_array( $dir, $ignore ) )
{
if( is_dir( $dir ) )
{
$title = file_get_contents( $dir.'/files/metadata/title.txt' );
$descrip = file_get_contents( $dir.'/files/metadata/description.txt' );
$icon = $dir.'/files/metadata/icon.png';
echo '<tr>';
echo '<td class="childIcon" rowspan="2">';
echo '<a href="'.$dir.'" class="child">';
echo '<img src="'.$icon.'" class="icon">';
echo '</a>';
echo '</td>';
echo '<td class="childIconLink">';
echo '<a href="'.$dir.'" class="child">'.$title.'</a>';
echo '</td>';
echo '</tr>';
echo '<tr>';
echo '<td class="childIconDescription">';
echo $descrip;
echo '</td>';
echo '</tr>';
}
}
}
echo '</table>';
echo '</div>';
echo '</nav>';
}
//-----------------------------------------------------------------------------
// video - draws a video player for $file of type mp4/aac (.m4v)
//-----------------------------------------------------------------------------
function video( $file )
{
$temp = explode( "/", $file );
$name = $temp[ count( $temp ) - 1 ];
$file = 'files/'.$file;
echo '<div class="video">';
echo '<video width="640" height="480" controls class="videoPlayer"';
echo '<source src="'.$file.'" type="video/mp4">';
echo '<a href="'.$file.'">'.$name.'</a>';
echo '</video>';
echo '</div>';
}
//-----------------------------------------------------------------------------
// href - draws a link pointing to $url with the name $title
//-----------------------------------------------------------------------------
function href( $url, $title )
{
echo '<a href="'.$url.'" target="_blank">'.$title.'</a>';
}
//-----------------------------------------------------------------------------
// download - draws a link for download pointing to $file with the name $title
//-----------------------------------------------------------------------------
function download( $file, $title )
{
$file = 'files/'.$file;
echo '<a href="'.$file.'" target="_blank">'.$title.'</a>';
}
//-----------------------------------------------------------------------------
// includeFile - includes $file onto the current page
//-----------------------------------------------------------------------------
function includeFile( $file )
{
$file = 'files/'.$file;
require( $file );
}
//-----------------------------------------------------------------------------
// embed - loads $url into a string then draws it onto the current page
//-----------------------------------------------------------------------------
function embed( $url )
{
$string = file_get_contents( $url );
echo $string;
}
//-----------------------------------------------------------------------------
// scrapeBody - scrapes the body out of an html document and echoes it locally
//-----------------------------------------------------------------------------
function scrapeBody( $url )
{
$pagestring = file_get_contents( $url );
$pagelength = strlen( $pagestring );
$bodyopen = strpos( $pagestring, '<body' );
$bodyclose = strpos( $pagestring, '</body>' );
$bodystring = substr( $pagestring, $bodyopen, ( $bodyclose - $pagelength ) );
$bodystring = substr( $bodystring, strpos( $bodystring, '>' ) + 1 );
echo $bodystring;
}
//-----------------------------------------------------------------------------
// breadcrumbs - draws links that lead back to the home page
//-----------------------------------------------------------------------------
function breadcrumbs()
{
global $userRoot;
$file = $_SERVER[ 'PHP_SELF' ];
$dir = pathinfo( $file, PATHINFO_DIRNAME );
$path = str_replace( $userRoot, '', $dir );
$dirs = explode( '/', $path );
$size = count( $dirs ) - 2;
$url = '';
echo '<div class="breadcrumbs">';
echo ' ';
echo '← ';
for( $i = $size; $i > 0 ; $i-- )
{
$url .= '../';
echo '<a href="'.$url.'">'.$dirs[ $i ].'</a> · ';
}
echo '<a href="'.$userRoot.'/home/search">search</a>';
echo '</div>';
}
//-----------------------------------------------------------------------------
// sitemap - draws a list of links to each page
//-----------------------------------------------------------------------------
function sitemap( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$ignore = array( '.', '..', '.DS_Store', 'files', 'Temp' );
if( $depth == 0 )
{
$dir = $docRoot.'/home';
echo '<a href="'.$userRoot.'/home">home</a><br>';
$depth = 1;
}
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
if( is_dir( $dir.'/'.$file ) && !in_array( $file, $ignore ) )
{
$url = $dir.'/'.$file;
$url = str_replace( $docRoot, $userRoot, $url );
echo str_repeat( ' ', ( $depth * 4 ) );
echo '<a href="'.$url.'">'.$file.'</a><br>';
sitemap( $dir.'/'.$file, ( $depth + 1 ) );
}
}
}
//-----------------------------------------------------------------------------
// sitemapEdit - draws a list of links to each page with query strings for edit
//-----------------------------------------------------------------------------
function sitemapEdit( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$ignore = array( '.', '..', '.DS_Store', 'files', 'Under Construction' );
if( $depth == 0 )
{
$dir = $docRoot.'/home';
echo '<a href="index.php?path='.$dir.'">home</a><br>';
$depth = 1;
}
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
$path = $dir.'/'.$file;
if( is_dir( $path ) && !in_array( $file, $ignore ) )
{
echo str_repeat( ' ', ( $depth * 4 ) );
echo '<a href="index.php?path='.$path.'">'.$file.'</a><br>';
sitemapEdit( $path, ( $depth + 1 ) );
}
}
}
//-----------------------------------------------------------------------------
// listFilesEdit - draws a list of files in each page's files directory
//-----------------------------------------------------------------------------
function listFilesEdit( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$ignore = array( '.', '..', '.DS_Store', 'metadata', 'content.php' );
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
$path = $dir.'/'.$file;
if( !in_array( $file, $ignore ) )
{
echo str_repeat( ' ', ( $depth * 4 ) );
if( is_dir( $path ) )
{
echo $file.'<br>';
listFilesEdit( $path, ( $depth + 1 ) );
}
else
{
$url = str_replace( $docRoot, $userRoot, $path );
echo '<a href="'.$url.'" target="_blank">'.$file.'</a><br>';
}
}
}
}
//-----------------------------------------------------------------------------
// googleMap - uses google maps api to draw a map centered at $lat, $lon
//-----------------------------------------------------------------------------
function googleMap( $lat, $lon )
{
echo '<div id="googleMapBox">';
echo ' <div id="googleMap">';
echo ' </div>';
echo '</div>';
echo '<script src="https://maps.googleapis.com/maps/api/js?v=3.exp&sensor=false"></script>';
echo '<script>';
echo ' function initialize()';
echo ' {';
echo ' var latLon = new google.maps.LatLng( '.$lat.', '.$lon.' );';
echo ' var mapOptions =';
echo ' {';
echo ' zoom: 10,';
echo ' center: latLon,';
echo ' disableDefaultUI: true,';
echo ' draggable: false,';
echo ' scrollwheel: false';
echo ' };';
echo ' var map = new google.maps.Map(document.getElementById( "googleMap" ), mapOptions );';
echo ' var marker = new google.maps.Marker( { position: latLon, map: map } );';
echo ' infowindow.open( map, marker );';
echo ' }';
echo ' initialize();';
echo '</script>';
}
//-----------------------------------------------------------------------------
// dropImage - drops a left floating image for text to flow around
//-----------------------------------------------------------------------------
function dropImage( $file, $width = '' )
{
$file = 'files/'.$file;
if( $width )
{
echo '<img src="'.$file.'" class="dropImage" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="dropImage">';
}
}
//-----------------------------------------------------------------------------
// dropPhoto - drops a left floating photo for text to flow around
//-----------------------------------------------------------------------------
function dropPhoto( $file, $width = '' )
{
$file = 'files/'.$file;
if( $width )
{
echo '<img src="'.$file.'" class="dropPhoto" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="dropPhoto">';
}
}
//-----------------------------------------------------------------------------
// dropIcon - drops a left floating icon for text to flow around
// circular icons should have a 2px black border
//-----------------------------------------------------------------------------
function dropIcon( $file = 'icon.png', $width = '' )
{
$file = 'files/metadata/'.$file;
if( $width )
{
echo '<img src="'.$file.'" class="dropPhoto" width="'.$width.'">';
}
else
{
echo '<img src="'.$file.'" class="dropIcon">';
}
}
//-----------------------------------------------------------------------------
// ls - draws a list of files
//-----------------------------------------------------------------------------
function ls( $dir = '', $depth = 0 )
{
global $docRoot;
global $userRoot;
$dir = 'files/'.$dir;
$ignore = array( '.', '..', '.DS_Store', 'metadata', 'content.php' );
$dh = opendir( $dir );
while( $file = readdir( $dh ) )
{
$files[] = $file;
}
closedir( $dh );
natsort( $files );
foreach( $files as $file )
{
$path = $dir.'/'.$file;
if( !in_array( $file, $ignore ) )
{
echo str_repeat( ' ', ( $depth * 4 ) );
if( is_dir( $path ) )
{
echo $file.'<br>';
listFilesEdit( $path, ( $depth + 1 ) );
}
else
{
$url = str_replace( $docRoot, $userRoot, $path );
echo '<a href="'.$url.'" target="_blank">'.$file.'</a><br>';
}
}
}
}
?>
utility.php
<?php
//-----------------------------------------------------------------------------
// varList - formatted var_dump()
//-----------------------------------------------------------------------------
function varList( $var )
{
echo '<pre>';
var_dump( $var );
echo '</pre>';
}
//-----------------------------------------------------------------------------
// hitCount - keeps track of visits to a particular page
//-----------------------------------------------------------------------------
function hitCount( $countFile )
{
$fh = fopen( $countFile, 'r' );
flock( $fh, LOCK_EX );
$hitsString = fgets( $fh );
fclose( $fh );
$hits = intval( $hitsString );
$fh = fopen( $countFile, 'w+' );
flock( $fh, LOCK_EX );
fwrite( $fh, $hits + 1 );
fflush( $fh );
fclose( $fh );
return $hits;
}
//-----------------------------------------------------------------------------
// visitorLog - writes an entry into the visitor log
//-----------------------------------------------------------------------------
function visitorLog()
{
global $docRoot;
$log = '/lib/visitor.log';
// $page = pathinfo( $_SERVER[ 'PHP_SELF' ], PATHINFO_DIRNAME );
$page = urldecode( $_SERVER[ 'REQUEST_URI' ] );
$agent = $_SERVER[ 'HTTP_USER_AGENT' ];
$ip = $_SERVER[ 'REMOTE_ADDR' ];
$name = gethostbyaddr( $ip );
$port = $_SERVER[ 'REMOTE_PORT' ];
$date = date( "l F jS Y g:i:sa" );
$epoch = microtime( 1 );
$fh = fopen( $docRoot.$log, 'a+' );
flock ( $fh, LOCK_EX );
fwrite( $fh, "Page : ".$page."\nVisitor : ".$ip." - ".$name."\nBrowser : ".$agent."\nDate : ".$date." - ".$epoch."\n\n" );
fflush( $fh );
fclose( $fh );
}
//-----------------------------------------------------------------------------
// userRootDir - returns name of user's URI root directory if page is there
//-----------------------------------------------------------------------------
function userRootDir()
{
$uri = $_SERVER[ 'REQUEST_URI' ];
$uid = posix_getpwuid( fileowner( __FILE__ ) );
$userDir = '/~'.$uid[ 'name' ];
if( !stristr( $uri, $userDir ) )
{
$userDir = '';
}
return $userDir;
}
//-----------------------------------------------------------------------------
// ipLocation - gets ip address location info from ipinfo.io
//-----------------------------------------------------------------------------
function ipLocation( $ip = '' )
{
if( $ip == '' )
{
$ip = $_SERVER[ 'REMOTE_ADDR' ];
}
$locString = file_get_contents( 'http://ipinfo.io/'.$ip );
$loc = json_decode( $locString, true );
$temp = explode( ',', $loc[ 'loc' ] );
$loc[ 'lat' ] = $temp[ 0 ];
$loc[ 'lon' ] = $temp[ 1 ];
return $loc;
}
?>
//-----------------------------------------------------------------------------
// varList - formatted var_dump()
//-----------------------------------------------------------------------------
function varList( $var )
{
echo '<pre>';
var_dump( $var );
echo '</pre>';
}
//-----------------------------------------------------------------------------
// hitCount - keeps track of visits to a particular page
//-----------------------------------------------------------------------------
function hitCount( $countFile )
{
$fh = fopen( $countFile, 'r' );
flock( $fh, LOCK_EX );
$hitsString = fgets( $fh );
fclose( $fh );
$hits = intval( $hitsString );
$fh = fopen( $countFile, 'w+' );
flock( $fh, LOCK_EX );
fwrite( $fh, $hits + 1 );
fflush( $fh );
fclose( $fh );
return $hits;
}
//-----------------------------------------------------------------------------
// visitorLog - writes an entry into the visitor log
//-----------------------------------------------------------------------------
function visitorLog()
{
global $docRoot;
$log = '/lib/visitor.log';
// $page = pathinfo( $_SERVER[ 'PHP_SELF' ], PATHINFO_DIRNAME );
$page = urldecode( $_SERVER[ 'REQUEST_URI' ] );
$agent = $_SERVER[ 'HTTP_USER_AGENT' ];
$ip = $_SERVER[ 'REMOTE_ADDR' ];
$name = gethostbyaddr( $ip );
$port = $_SERVER[ 'REMOTE_PORT' ];
$date = date( "l F jS Y g:i:sa" );
$epoch = microtime( 1 );
$fh = fopen( $docRoot.$log, 'a+' );
flock ( $fh, LOCK_EX );
fwrite( $fh, "Page : ".$page."\nVisitor : ".$ip." - ".$name."\nBrowser : ".$agent."\nDate : ".$date." - ".$epoch."\n\n" );
fflush( $fh );
fclose( $fh );
}
//-----------------------------------------------------------------------------
// userRootDir - returns name of user's URI root directory if page is there
//-----------------------------------------------------------------------------
function userRootDir()
{
$uri = $_SERVER[ 'REQUEST_URI' ];
$uid = posix_getpwuid( fileowner( __FILE__ ) );
$userDir = '/~'.$uid[ 'name' ];
if( !stristr( $uri, $userDir ) )
{
$userDir = '';
}
return $userDir;
}
//-----------------------------------------------------------------------------
// ipLocation - gets ip address location info from ipinfo.io
//-----------------------------------------------------------------------------
function ipLocation( $ip = '' )
{
if( $ip == '' )
{
$ip = $_SERVER[ 'REMOTE_ADDR' ];
}
$locString = file_get_contents( 'http://ipinfo.io/'.$ip );
$loc = json_decode( $locString, true );
$temp = explode( ',', $loc[ 'loc' ] );
$loc[ 'lat' ] = $temp[ 0 ];
$loc[ 'lon' ] = $temp[ 1 ];
return $loc;
}
?>
view.php
<?php
require( 'main.php' );
if( isset( $_REQUEST[ 'image' ] ) )
{
$image = $_REQUEST[ 'image' ];
$image = stripslashes( $image );
}
else
{
$image = '';
}
$title = pathinfo( $image, PATHINFO_FILENAME );
$ext = pathinfo( $image, PATHINFO_EXTENSION );
$dir = pathinfo( $image, PATHINFO_DIRNAME );
$file = $title.'.'.$ext;
$description = $title.' photograph';
$keywords = 'cooper, baker, cooperbaker, '.$file.', '.$title.', '.$ext.', photo, photograph, image, picture, graphic, download, stock, press, print, high, resolution, res, hi-res, high-res, purchase, buy, publish, publication';
$copyright = '(c)(p) '.date( 'Y' ).' Cooper Baker - All Rights Reserved';
$timestamp = '<!-- This page was automatically generated on '.date( 'l F jS Y' ).' at '.date( 'g:i:sa' )." -->\n";
$stylesheet = $userRoot.'/lib/style.php';
$favicon = $userRoot.'/lib/images/favicon.png';
function prevNext( $image )
{
global $docRoot;
global $userRoot;
global $dir;
$dir = pathinfo( $image, PATHINFO_DIRNAME );
$imgFile = pathinfo( $image, PATHINFO_BASENAME );
$images;
$i = 0;
$path = str_replace( $userRoot, '', $dir );
if( $dh = opendir( $docRoot.$path ) )
{
while( false !== ( $file = readdir( $dh ) ) )
{
$type = pathinfo( $file, PATHINFO_EXTENSION );
if( ( $type == 'jpg' )
|| ( $type == 'JPG' )
|| ( $type == 'jpeg' )
|| ( $type == 'JPEG' )
|| ( $type == 'gif' )
|| ( $type == 'GIF' )
|| ( $type == 'png' )
|| ( $type == 'PNG' )
|| ( $type == 'tif' )
|| ( $type == 'TIF' )
|| ( $type == 'tiff' )
|| ( $type == 'TIFF' )
|| ( $type == 'svg' )
|| ( $type == 'SVG' )
|| ( $type == 'bmp' )
|| ( $type == 'BMP' ) )
{
$images[ $i ] = $file;
$i++;
}
}
closedir( $dh );
}
$numImages = count( $images );
natsort( $images );
$images = array_values( $images );
if( $numImages > 1 )
{
$imgIndex = array_search( $imgFile, $images );
$prevImage = $images[ ( $imgIndex - 1 + $numImages ) % $numImages ];
$nextImage = $images[ ( $imgIndex + 1 ) % $numImages ];
echo '<a href="'.$userRoot.'/lib/view.php?image='.$dir.'/'.$prevImage.'" class="view">←</a>';
echo ' · ';
echo '<a href="'.$userRoot.'/lib/view.php?image='.$dir.'/'.$nextImage.'" class="view">→</a>';
}
}
?>
<!DOCTYPE html>
<html>
<head>
<?php echo $timestamp; ?>
<meta charset="UTF-8">
<meta name="author" content="Cooper Baker">
<meta name="copyright" content="<?php echo $copyright; ?>">
<meta name="description" content="<?php echo $description; ?>">
<meta name="keywords" content="<?php echo $keywords; ?>">
<title><?php echo $title; ?></title>
<link rel="stylesheet" href="<?php echo $stylesheet; ?>" type="text/css">
<link rel="icon" href="<?php echo $favicon; ?>" type="image/x-icon" type="text/css">
<meta name="robots" content="index, follow">
<meta name="googlebot" content="index, follow">
<?php require( $docRoot.'/lib/google.verification.php' ); ?>
</head>
<body>
<div id="menu">
<div id="menuphoto">
<?php echo $title; ?>
</div>
</div>
<br>
<div id="contentView">
<a href="<?php echo $image; ?>" alt="<?php echo $title; ?>" target="_blank">
<?php
$type = pathinfo( $image, PATHINFO_EXTENSION );
if( ( $type == 'png' ) || ( $type == 'PNG' ) )
{
echo '<img src="'.$image.'" alt="'.$title.'" class="photoView" style="border-style: none;">';
}
else
{
echo '<img src="'.$image.'" alt="'.$title.'" class="photoView">';
}
?>
</a>
<div id="photoPrevNext">
<?php prevNext( $image ); ?>
</div>
</div>
<div id="copyright">
<center>
©<?php echo date( 'Y' ); ?> Cooper Baker
·
All Rights Reserved
·
High-Res Copy Available For Purchase
·
cooperbaker@gmail·com
</center>
</div>
<?php require( $docRoot.'/lib/google.analytics.php' ); ?>
</body>
</html>
<?php visitorLog() ?>
require( 'main.php' );
if( isset( $_REQUEST[ 'image' ] ) )
{
$image = $_REQUEST[ 'image' ];
$image = stripslashes( $image );
}
else
{
$image = '';
}
$title = pathinfo( $image, PATHINFO_FILENAME );
$ext = pathinfo( $image, PATHINFO_EXTENSION );
$dir = pathinfo( $image, PATHINFO_DIRNAME );
$file = $title.'.'.$ext;
$description = $title.' photograph';
$keywords = 'cooper, baker, cooperbaker, '.$file.', '.$title.', '.$ext.', photo, photograph, image, picture, graphic, download, stock, press, print, high, resolution, res, hi-res, high-res, purchase, buy, publish, publication';
$copyright = '(c)(p) '.date( 'Y' ).' Cooper Baker - All Rights Reserved';
$timestamp = '<!-- This page was automatically generated on '.date( 'l F jS Y' ).' at '.date( 'g:i:sa' )." -->\n";
$stylesheet = $userRoot.'/lib/style.php';
$favicon = $userRoot.'/lib/images/favicon.png';
function prevNext( $image )
{
global $docRoot;
global $userRoot;
global $dir;
$dir = pathinfo( $image, PATHINFO_DIRNAME );
$imgFile = pathinfo( $image, PATHINFO_BASENAME );
$images;
$i = 0;
$path = str_replace( $userRoot, '', $dir );
if( $dh = opendir( $docRoot.$path ) )
{
while( false !== ( $file = readdir( $dh ) ) )
{
$type = pathinfo( $file, PATHINFO_EXTENSION );
if( ( $type == 'jpg' )
|| ( $type == 'JPG' )
|| ( $type == 'jpeg' )
|| ( $type == 'JPEG' )
|| ( $type == 'gif' )
|| ( $type == 'GIF' )
|| ( $type == 'png' )
|| ( $type == 'PNG' )
|| ( $type == 'tif' )
|| ( $type == 'TIF' )
|| ( $type == 'tiff' )
|| ( $type == 'TIFF' )
|| ( $type == 'svg' )
|| ( $type == 'SVG' )
|| ( $type == 'bmp' )
|| ( $type == 'BMP' ) )
{
$images[ $i ] = $file;
$i++;
}
}
closedir( $dh );
}
$numImages = count( $images );
natsort( $images );
$images = array_values( $images );
if( $numImages > 1 )
{
$imgIndex = array_search( $imgFile, $images );
$prevImage = $images[ ( $imgIndex - 1 + $numImages ) % $numImages ];
$nextImage = $images[ ( $imgIndex + 1 ) % $numImages ];
echo '<a href="'.$userRoot.'/lib/view.php?image='.$dir.'/'.$prevImage.'" class="view">←</a>';
echo ' · ';
echo '<a href="'.$userRoot.'/lib/view.php?image='.$dir.'/'.$nextImage.'" class="view">→</a>';
}
}
?>
<!DOCTYPE html>
<html>
<head>
<?php echo $timestamp; ?>
<meta charset="UTF-8">
<meta name="author" content="Cooper Baker">
<meta name="copyright" content="<?php echo $copyright; ?>">
<meta name="description" content="<?php echo $description; ?>">
<meta name="keywords" content="<?php echo $keywords; ?>">
<title><?php echo $title; ?></title>
<link rel="stylesheet" href="<?php echo $stylesheet; ?>" type="text/css">
<link rel="icon" href="<?php echo $favicon; ?>" type="image/x-icon" type="text/css">
<meta name="robots" content="index, follow">
<meta name="googlebot" content="index, follow">
<?php require( $docRoot.'/lib/google.verification.php' ); ?>
</head>
<body>
<div id="menu">
<div id="menuphoto">
<?php echo $title; ?>
</div>
</div>
<br>
<div id="contentView">
<a href="<?php echo $image; ?>" alt="<?php echo $title; ?>" target="_blank">
<?php
$type = pathinfo( $image, PATHINFO_EXTENSION );
if( ( $type == 'png' ) || ( $type == 'PNG' ) )
{
echo '<img src="'.$image.'" alt="'.$title.'" class="photoView" style="border-style: none;">';
}
else
{
echo '<img src="'.$image.'" alt="'.$title.'" class="photoView">';
}
?>
</a>
<div id="photoPrevNext">
<?php prevNext( $image ); ?>
</div>
</div>
<div id="copyright">
<center>
©<?php echo date( 'Y' ); ?> Cooper Baker
·
All Rights Reserved
·
High-Res Copy Available For Purchase
·
cooperbaker@gmail·com
</center>
</div>
<?php require( $docRoot.'/lib/google.analytics.php' ); ?>
</body>
</html>
<?php visitorLog() ?>
style.php
<?php
header( "Content-type: text/css; charset: UTF-8" );
// Background
//-------------------------------------------------------------------------
$bimg = 'url( "images/paper.png" )';
// Colors
//-------------------------------------------------------------------------
$fgnd = '#333333'; // foreground
$bgnd = '#FFFEFD'; // background
$hglt = '#cc2222'; // highlight
$link = '#cc2222'; // link
$hlnk = '#ff3333'; // hover link
// Fonts
//-------------------------------------------------------------------------
$type = 'Georgia, Times, "Times New Roman", serif';
$mono = 'Menlo, monospace';
// Show - shows dotted red border of containers
//-------------------------------------------------------------------------
function show( $draw = 1 ){ if( $draw ) echo( 'border: 1px dotted red;' ); }
?>
body
{
font-family: <?php echo $type ?>;
color: <?php echo $fgnd ?>;
background: <?php echo $bgnd ?>;
background-image: <?php echo $bimg ?>;
font-size: 100%;
margin: 0px;
}
img
{
border: none;
}
a
{
text-decoration: none;
}
a.child
{
font-size: 1.3em;
letter-spacing: 0.03em;
}
a.breadcrumbLink
{
font-size: 0.8em;
letter-spacing: 0.01em;
vertical-align: bottom;
}
a:link { color: <?php echo $link ?>; }
a:hover { color: <?php echo $hlnk ?>; }
a:active { color: <?php echo $hlnk ?>; }
a:visited{ color: <?php echo $link ?>; }
a.view:link { color: <?php echo $fgnd ?>; }
a.view:hover { color: <?php echo $link ?>; }
a.view:active { color: <?php echo $link ?>; }
a.view:visited{ color: <?php echo $fgnd ?>; }
#frontPage
{
position: absolute;
overflow-y: auto;
overflow-x: auto;
top: 0em;
left: 0em;
right: 0em;
bottom: 0em;
}
#menu
{
font-size: 2em;
letter-spacing: 0.05em;
position: absolute;
overflow: hidden;
white-space: nowrap;
top: 0px;
left: 0px;
right: 0px;
height: 1em;
padding-top: 0.2em;
padding-bottom: 0.3em;
padding-left: 1.5em;
padding-right: 1.5em;
}
#menuleft
{
display: inline;
float: left;
}
#menuright
{
text-align: right;
}
#menuphoto
{
text-align: center;
}
#content
{
font-size: 1.2em;
letter-spacing: 0.02em;
text-align: justify;
background-image: <?php echo $bimg ?>;
background-attachment: local;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
top: 2.6em;
left: 0px;
right: 0px;
bottom: 0.8em;
padding-top: 1.2em;
padding-left: 2.6em;
padding-right: 2.6em;
border-color: <?php echo $fgnd ?>;
border-style: solid;
border-width: 0px;
border-top-width: 0.2em;
border-bottom-width: 0.1em;
}
#contentView
{
font-size: 1.2em;
letter-spacing: 0.02em;
background-image: <?php echo $bimg ?>;
background-attachment: local;
position: absolute;
text-align: center;
overflow-y: auto;
overflow-x: auto;
top: 2.666666em;
left: 0px;
right: 0px;
bottom: 0.8em;
padding-left: 1.3em;
padding-right: 1.3em;
padding-top: 2.6em;
padding-bottom: 2.6em;
border-color: <?php echo $fgnd ?>;
border-style: solid;
border-width: 0px;
border-top-width: 0.2em;
border-bottom-width: 0.1em;
}
.photoView
{
position: absolute;
left: 0;
right: 0;
top: 0;
bottom: 0;
margin: auto;
height: auto;
width: auto;
max-width: 95%;
max-height: 95%;
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
#photoPrevNext
{
position: absolute;
left: 0;
right: 0;
bottom: 0.25em;
margin-left: auto;
margin-right: auto;
font-size: 2em;
}
#copyright
{
font-size: 0.7em;
letter-spacing: 0em;
padding: 0.1em;
position: fixed;
bottom: 0em;
left: 0em;
right: 0em;
text-align: right;
background-image: <?php echo $bimg ?>;
}
.ruler
{
font-size: 1.3em;
letter-spacing: 0.03em;
position: relative;
clear: both;
margin-top: 0.1em;
margin-bottom: 0.4em;
margin-left: -1em;
margin-right: -1em;
padding-left: 1em;
padding-right: 1em;
padding-bottom: 0.1em;
border-color: <?php echo $hglt ?>;
border-style: solid;
border-width: 0px;
border-bottom-width: 2px;
}
.bigText
{
font-size: 1.15em;
letter-spacing: 0.01em;
}
.gallery
{
position: relative;
overflow: hidden;
text-align: center;
padding-top: 0.5em;
padding-bottom: 0.5em;
}
.thumbBox
{
display: inline-block;
overflow: hidden;
width: 25%;
text-align: center;
vertical-align: middle;
}
.thumb
{
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.code
{
font-size: 0.85em;
position: relative;
}
.image
{
position: relative;
text-align: center;
}
.photoBox
{
position: relative;
text-align: center;
}
.photo
{
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.audio
{
position: relative;
}
.audioPlayer
{
display: inline-block;
width: 100%;
}
.children
{
position: relative;
overflow: hidden;
text-align: left;
}
.childTable
{
margin: 0px;
padding: 0em;
border: 0px;
}
.icon
{
width: 52px;
}
.childIcon
{
text-align: center;
vertical-align: top;
width: 52px;
height: 52px;
padding-top: 0.125em;
padding-left: 0.666666em;
padding-right: 0.666666em;
<?php show( 0 ) ?>
}
.childIconLink
{
text-align: left;
vertical-align: top;
padding-top: 0em;
padding-bottom: 0em;
}
.childIconDescription
{
text-align: left;
vertical-align: top;
font-size: 1.1em;
letter-spacing: 0.03em;
padding-bottom: 1em;
}
.childLink
{
text-align: right;
vertical-align: top;
padding: 0.2em;
padding-left: 0em;
}
.childDescription
{
text-align: left;
vertical-align: top;
font-size: 1.3em;
letter-spacing: 0.03em;
padding: 0.2em;
padding-right: 0em;
padding-left: 0.5em;
}
.video
{
position: relative;
text-align: center;
}
.videoPlayer
{
display: inline-block;
}
.breadcrumbs
{
font-size: 0.6em;
letter-spacing: 0.02em;
position: relative;
display: inline;
}
input
{
font-family: <?php echo $mono ?>;
border-style: solid;
border-width: 1px;
border-color: <?php echo $fgnd ?>;
padding: 0.25em;
}
textarea
{
resize: none;
font-family: <?php echo $mono ?>;
border-style: solid;
border-width: 1px;
border-color: <?php echo $fgnd ?>;
padding: 0.25em;
}
#editFieldTitle
{
width: 53em;
height: 1.1em;
overflow: hidden;
}
#editFieldDescription
{
width: 53em;
height: 1.1em;
overflow: hidden;
}
#editFieldKeywords
{
width: 53em;
height: 3.5em;
overflow: hidden;
}
#editFieldContent
{
width: 53em;
height: 64em;
overflow-y: auto;
}
#editFieldContentAceBox
{
position: relative;
top: 0;
left: 0;
right: 0;
bottom: 0;
}
#editFieldContentAce
{
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
font-family: <?php echo $mono ?>;
font-size: 0.66em;
font-weight: normal;
border-style: solid;
border-width: 1px;
border-color: <?php echo $fgnd ?>;
}
#editCursorPosition
{
float: left;
}
#siteMapEdit
{
font-size: 1em;
letter-spacing: 0em;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
white-space: nowrap;
top: 0px;
left: 1em;
width: 10.666666em;
bottom: 0px;
padding-top: 0.5em;
padding-left: 1em;
padding-right: 2.333333em;
}
#editFields
{
font-size: 1em;
letter-spacing: 0em;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
top: 0px;
left: 15em;
right: 16em;
bottom: 0px;
padding-top: 0.5em;
padding-left: 1em;
padding-right: 1em;
}
#files
{
font-size: 1em;
letter-spacing: 0em;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
white-space: nowrap;
top: 0px;
right: 0em;
width: 11.666666em;
bottom: 0px;
padding-top: 0.5em;
padding-left: 1em;
padding-right: 2.333333em;
}
.editFieldName
{
text-align: right;
vertical-align: top;
}
.editFieldInput
{
text-align: left;
vertical-align: top;
}
#googleMapBox
{
position: relative;
text-align: center;
}
#googleMap
{
width: 800px;
height: 300px;
display: inline-block;
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.dropImage
{
float: left;
margin-top: 0.125em;
margin-right: 1em;
margin-bottom: 0.75em;
}
.dropPhoto
{
float: left;
margin-top: 0.125em;
margin-right: 1em;
margin-bottom: 0.75em;
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.dropIcon
{
float: left;
margin-top: 0.125em;
margin-right: 1em;
margin-bottom: 0.75em;
}
header( "Content-type: text/css; charset: UTF-8" );
// Background
//-------------------------------------------------------------------------
$bimg = 'url( "images/paper.png" )';
// Colors
//-------------------------------------------------------------------------
$fgnd = '#333333'; // foreground
$bgnd = '#FFFEFD'; // background
$hglt = '#cc2222'; // highlight
$link = '#cc2222'; // link
$hlnk = '#ff3333'; // hover link
// Fonts
//-------------------------------------------------------------------------
$type = 'Georgia, Times, "Times New Roman", serif';
$mono = 'Menlo, monospace';
// Show - shows dotted red border of containers
//-------------------------------------------------------------------------
function show( $draw = 1 ){ if( $draw ) echo( 'border: 1px dotted red;' ); }
?>
body
{
font-family: <?php echo $type ?>;
color: <?php echo $fgnd ?>;
background: <?php echo $bgnd ?>;
background-image: <?php echo $bimg ?>;
font-size: 100%;
margin: 0px;
}
img
{
border: none;
}
a
{
text-decoration: none;
}
a.child
{
font-size: 1.3em;
letter-spacing: 0.03em;
}
a.breadcrumbLink
{
font-size: 0.8em;
letter-spacing: 0.01em;
vertical-align: bottom;
}
a:link { color: <?php echo $link ?>; }
a:hover { color: <?php echo $hlnk ?>; }
a:active { color: <?php echo $hlnk ?>; }
a:visited{ color: <?php echo $link ?>; }
a.view:link { color: <?php echo $fgnd ?>; }
a.view:hover { color: <?php echo $link ?>; }
a.view:active { color: <?php echo $link ?>; }
a.view:visited{ color: <?php echo $fgnd ?>; }
#frontPage
{
position: absolute;
overflow-y: auto;
overflow-x: auto;
top: 0em;
left: 0em;
right: 0em;
bottom: 0em;
}
#menu
{
font-size: 2em;
letter-spacing: 0.05em;
position: absolute;
overflow: hidden;
white-space: nowrap;
top: 0px;
left: 0px;
right: 0px;
height: 1em;
padding-top: 0.2em;
padding-bottom: 0.3em;
padding-left: 1.5em;
padding-right: 1.5em;
}
#menuleft
{
display: inline;
float: left;
}
#menuright
{
text-align: right;
}
#menuphoto
{
text-align: center;
}
#content
{
font-size: 1.2em;
letter-spacing: 0.02em;
text-align: justify;
background-image: <?php echo $bimg ?>;
background-attachment: local;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
top: 2.6em;
left: 0px;
right: 0px;
bottom: 0.8em;
padding-top: 1.2em;
padding-left: 2.6em;
padding-right: 2.6em;
border-color: <?php echo $fgnd ?>;
border-style: solid;
border-width: 0px;
border-top-width: 0.2em;
border-bottom-width: 0.1em;
}
#contentView
{
font-size: 1.2em;
letter-spacing: 0.02em;
background-image: <?php echo $bimg ?>;
background-attachment: local;
position: absolute;
text-align: center;
overflow-y: auto;
overflow-x: auto;
top: 2.666666em;
left: 0px;
right: 0px;
bottom: 0.8em;
padding-left: 1.3em;
padding-right: 1.3em;
padding-top: 2.6em;
padding-bottom: 2.6em;
border-color: <?php echo $fgnd ?>;
border-style: solid;
border-width: 0px;
border-top-width: 0.2em;
border-bottom-width: 0.1em;
}
.photoView
{
position: absolute;
left: 0;
right: 0;
top: 0;
bottom: 0;
margin: auto;
height: auto;
width: auto;
max-width: 95%;
max-height: 95%;
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
#photoPrevNext
{
position: absolute;
left: 0;
right: 0;
bottom: 0.25em;
margin-left: auto;
margin-right: auto;
font-size: 2em;
}
#copyright
{
font-size: 0.7em;
letter-spacing: 0em;
padding: 0.1em;
position: fixed;
bottom: 0em;
left: 0em;
right: 0em;
text-align: right;
background-image: <?php echo $bimg ?>;
}
.ruler
{
font-size: 1.3em;
letter-spacing: 0.03em;
position: relative;
clear: both;
margin-top: 0.1em;
margin-bottom: 0.4em;
margin-left: -1em;
margin-right: -1em;
padding-left: 1em;
padding-right: 1em;
padding-bottom: 0.1em;
border-color: <?php echo $hglt ?>;
border-style: solid;
border-width: 0px;
border-bottom-width: 2px;
}
.bigText
{
font-size: 1.15em;
letter-spacing: 0.01em;
}
.gallery
{
position: relative;
overflow: hidden;
text-align: center;
padding-top: 0.5em;
padding-bottom: 0.5em;
}
.thumbBox
{
display: inline-block;
overflow: hidden;
width: 25%;
text-align: center;
vertical-align: middle;
}
.thumb
{
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.code
{
font-size: 0.85em;
position: relative;
}
.image
{
position: relative;
text-align: center;
}
.photoBox
{
position: relative;
text-align: center;
}
.photo
{
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.audio
{
position: relative;
}
.audioPlayer
{
display: inline-block;
width: 100%;
}
.children
{
position: relative;
overflow: hidden;
text-align: left;
}
.childTable
{
margin: 0px;
padding: 0em;
border: 0px;
}
.icon
{
width: 52px;
}
.childIcon
{
text-align: center;
vertical-align: top;
width: 52px;
height: 52px;
padding-top: 0.125em;
padding-left: 0.666666em;
padding-right: 0.666666em;
<?php show( 0 ) ?>
}
.childIconLink
{
text-align: left;
vertical-align: top;
padding-top: 0em;
padding-bottom: 0em;
}
.childIconDescription
{
text-align: left;
vertical-align: top;
font-size: 1.1em;
letter-spacing: 0.03em;
padding-bottom: 1em;
}
.childLink
{
text-align: right;
vertical-align: top;
padding: 0.2em;
padding-left: 0em;
}
.childDescription
{
text-align: left;
vertical-align: top;
font-size: 1.3em;
letter-spacing: 0.03em;
padding: 0.2em;
padding-right: 0em;
padding-left: 0.5em;
}
.video
{
position: relative;
text-align: center;
}
.videoPlayer
{
display: inline-block;
}
.breadcrumbs
{
font-size: 0.6em;
letter-spacing: 0.02em;
position: relative;
display: inline;
}
input
{
font-family: <?php echo $mono ?>;
border-style: solid;
border-width: 1px;
border-color: <?php echo $fgnd ?>;
padding: 0.25em;
}
textarea
{
resize: none;
font-family: <?php echo $mono ?>;
border-style: solid;
border-width: 1px;
border-color: <?php echo $fgnd ?>;
padding: 0.25em;
}
#editFieldTitle
{
width: 53em;
height: 1.1em;
overflow: hidden;
}
#editFieldDescription
{
width: 53em;
height: 1.1em;
overflow: hidden;
}
#editFieldKeywords
{
width: 53em;
height: 3.5em;
overflow: hidden;
}
#editFieldContent
{
width: 53em;
height: 64em;
overflow-y: auto;
}
#editFieldContentAceBox
{
position: relative;
top: 0;
left: 0;
right: 0;
bottom: 0;
}
#editFieldContentAce
{
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
font-family: <?php echo $mono ?>;
font-size: 0.66em;
font-weight: normal;
border-style: solid;
border-width: 1px;
border-color: <?php echo $fgnd ?>;
}
#editCursorPosition
{
float: left;
}
#siteMapEdit
{
font-size: 1em;
letter-spacing: 0em;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
white-space: nowrap;
top: 0px;
left: 1em;
width: 10.666666em;
bottom: 0px;
padding-top: 0.5em;
padding-left: 1em;
padding-right: 2.333333em;
}
#editFields
{
font-size: 1em;
letter-spacing: 0em;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
top: 0px;
left: 15em;
right: 16em;
bottom: 0px;
padding-top: 0.5em;
padding-left: 1em;
padding-right: 1em;
}
#files
{
font-size: 1em;
letter-spacing: 0em;
position: absolute;
overflow-y: auto;
overflow-x: hidden;
white-space: nowrap;
top: 0px;
right: 0em;
width: 11.666666em;
bottom: 0px;
padding-top: 0.5em;
padding-left: 1em;
padding-right: 2.333333em;
}
.editFieldName
{
text-align: right;
vertical-align: top;
}
.editFieldInput
{
text-align: left;
vertical-align: top;
}
#googleMapBox
{
position: relative;
text-align: center;
}
#googleMap
{
width: 800px;
height: 300px;
display: inline-block;
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.dropImage
{
float: left;
margin-top: 0.125em;
margin-right: 1em;
margin-bottom: 0.75em;
}
.dropPhoto
{
float: left;
margin-top: 0.125em;
margin-right: 1em;
margin-bottom: 0.75em;
border-width: 1px;
border-style: solid;
border-color: <?php echo $fgnd ?>;
}
.dropIcon
{
float: left;
margin-top: 0.125em;
margin-right: 1em;
margin-bottom: 0.75em;
}